How to add React Component to ASP.Net Core Project
On the previous blog, we have discussed on how to create an ASP.Net Core Project with React JS.
Now that we know how to create a project, we will now go ahead and create a new Component to the project.
To get started, go to the “Components” folder and add a new JS file.
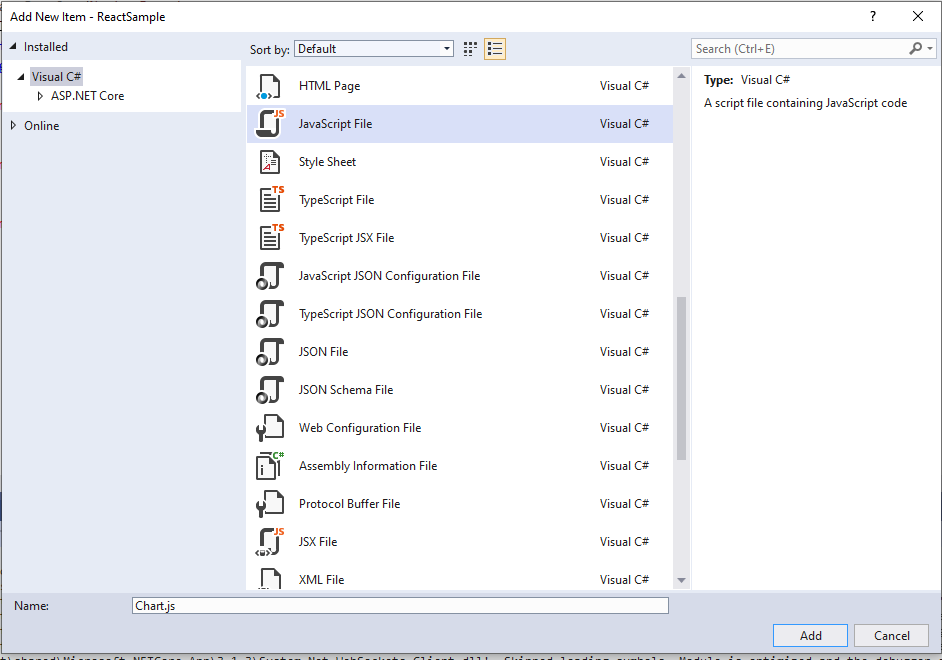
On the created js file, paste the following code:
import React, { Component } from 'react';
export class Chart extends Component {
render() {
return (
<div>
<h1>Chart JS</h1>
</div>
);
}
}
The render function contains the html part of your component. Now to be able to add an end point to your component. Go to App.js and add the following code:
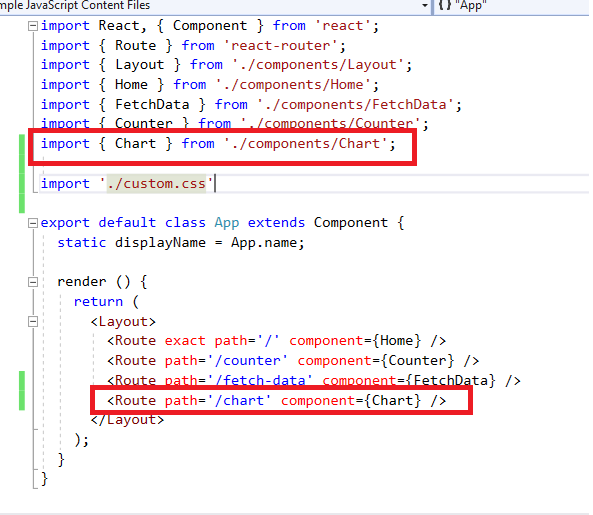
Now, when you go to your [project-url]/chart, you will now see that the new component renders on the page.

After creating an endpoint to your component, you’ll notice that you will still need to add an option on the menu to access the component. To do that open NavMenu.js and add this line of code.
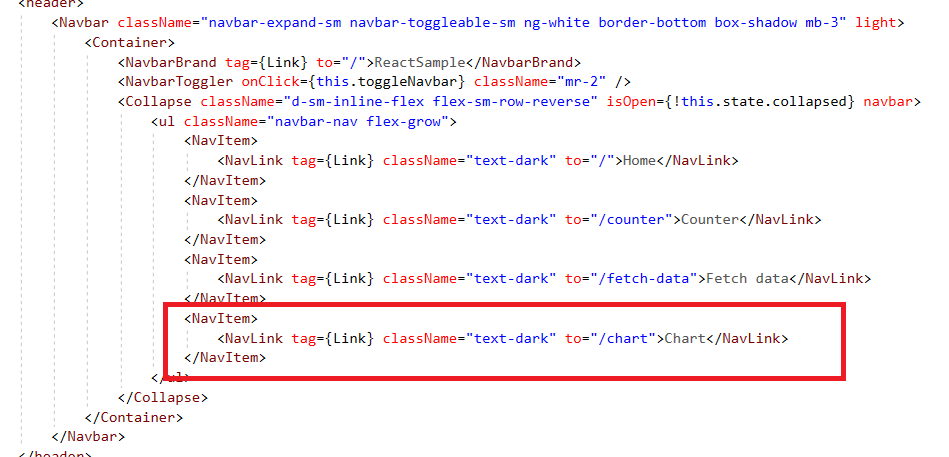
Now refresh your browser, you’ll notice that there is additional menu added on the nvaigation. When you click on it, you will be redirected on the new component end-point.
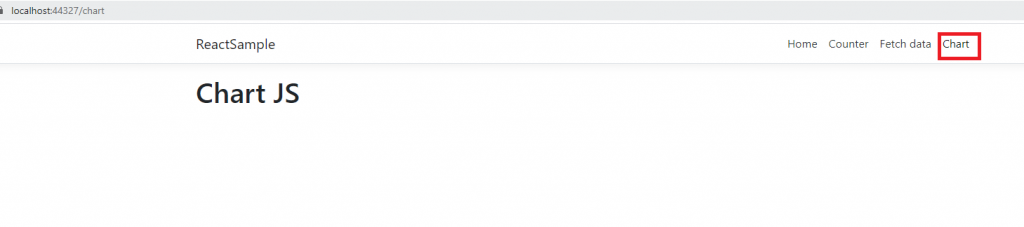
Now we already know how to add new components, create an end-point and add it on the navigation menu. The next thing that we’re going to do is how to render Chart.js into a React Component.